【Excel・VBA】ファイルを一括でPDF化する方法(サンプルコードあり)
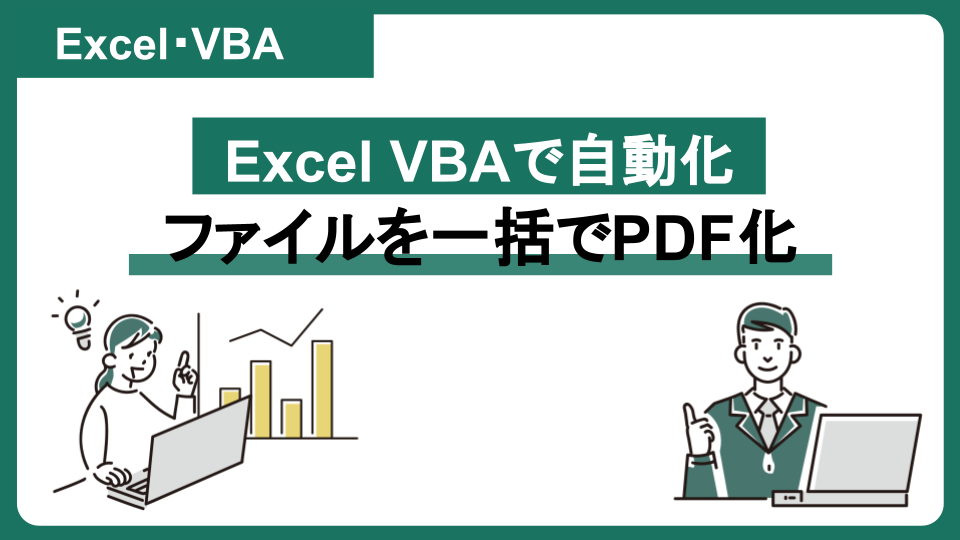
こんにちは!
今回は、複数のファイルを一括でPDF化する方法をご紹介します。
VBAで、ファイルを一括でPDF化する方法が分かる
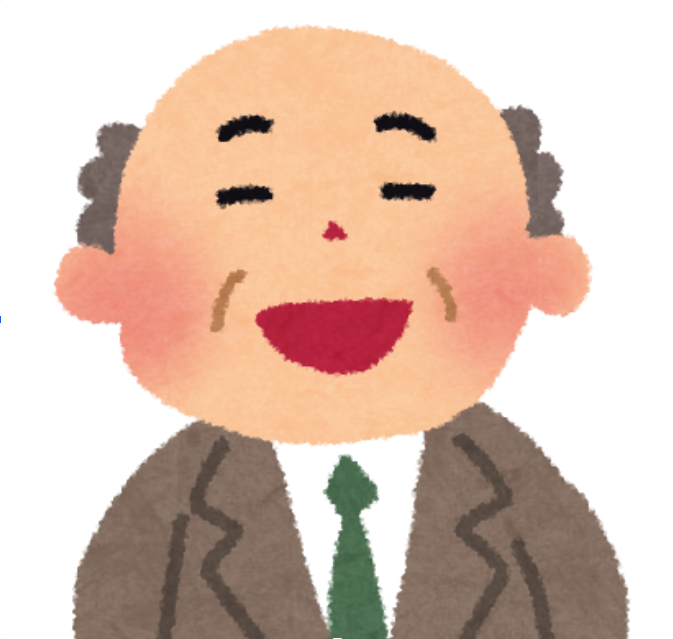
社会人くん、このフォルダに入っているExcelを全部PDFにしてくれるかな?
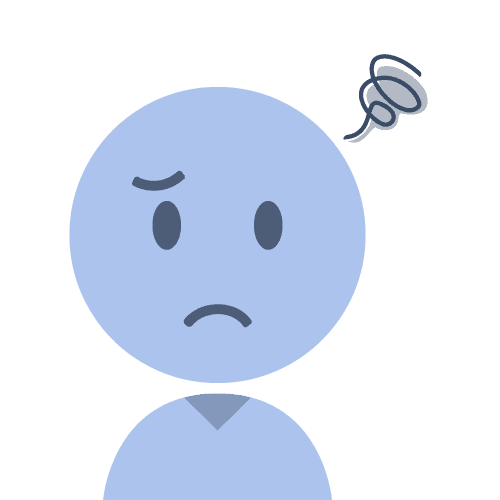
分かりました部長、、、、
って、ファイルが100個もあるじゃないですか!!
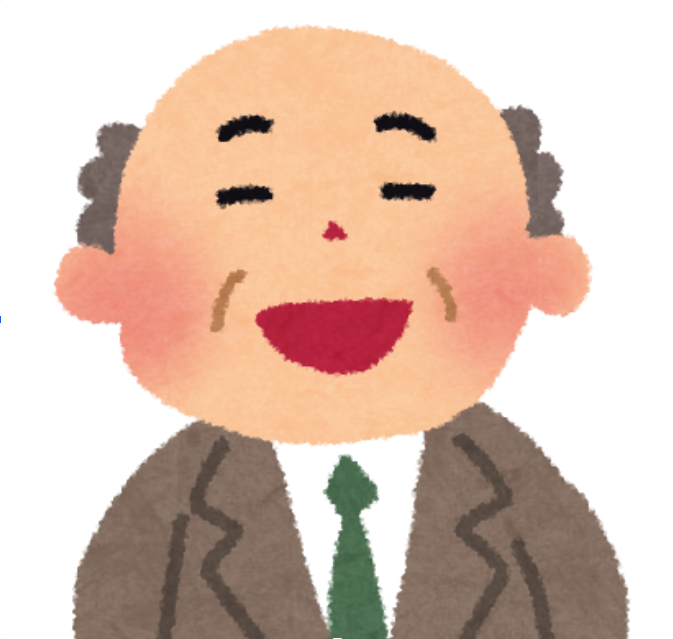
大変だと思うけど頑張ってね〜
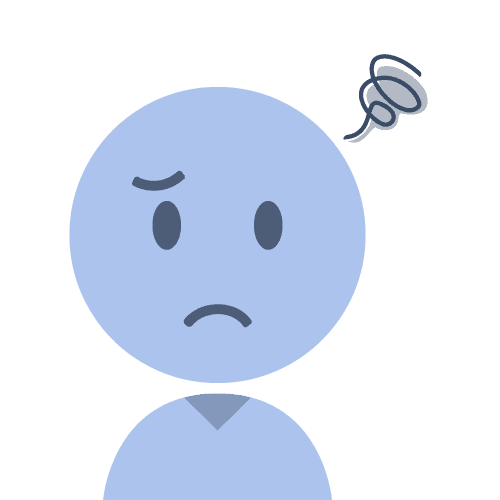
全てPDF化するのにどれくらいかかるんだ、、、
はじめに
普段の業務で、大量のファイルをPDF化する場面ってありますよね。
手作業で処理することもできますが、ファイル数が増えてくると時間がかかります。特に、定期的にこのような業務が発生している場合には、「この作業が一瞬でできならな〜」と考えたことがあるのではないでしょうか。
このような悩みは、VBAによる自動化で解決できます。次の章から、ファイルを一括でPDF化するコードを紹介します。
ファイルをPDF化するコード
ファイルをPDF化するコードは下記のとおりです。
1Sub Convert_to_PDF()
2 Dim strDirPath As String
3 strDirPath = Search_Directory() 'フォルダの選択
4 If Len(strDirPath) = 0 Then Exit Sub
5 Call Make_Dir(strDirPath, "\PDF") 'フォルダ作成
6 Call Search_Files(strDirPath)
7 MsgBox ("pdfファイル作成が完了しました")
8End Sub
9
10Private Function Search_Directory() As String 'フォルダの選択
11 With Application.FileDialog(msoFileDialogFolderPicker)
12 If .Show = True Then Search_Directory = .SelectedItems(1)
13 End With
14End Function
15
16Private Sub Make_Dir(ByVal Path As String, ByVal Folder_name As String)
17 If Dir(Path & Folder_name, vbDirectory) = "" Then 'フォルダの存在確認
18 MkDir Path & Folder_name 'フォルダ作成
19 End If
20End Sub
21
22Private Sub Search_Files(ByVal Path As String)
23 Dim strFile As String, strET As String
24 strFile = Dir(Path & "\" & "*.*") 'ファイルの存在確認
25 Application.ScreenUpdating = False
26 Do Until strFile = ""
27 If ThisWorkbook.FullName <> Path & "\" & strFile Then
28 Call Conv_PDF(Path, "\" & strFile)
29 End If
30 strFile = Dir() '次のファイル確認
31 Loop
32 Application.ScreenUpdating = True
33End Sub
34
35Private Function Get_Extension(ByVal Path As String) As String '拡張子を取得
36 Dim i As Long
37 i = InStrRev(Path, ".", -1, vbTextCompare)
38 If i = 0 Then Exit Function
39 Get_Extension = Mid$(Path, i + 1)
40End Function
41
42Private Sub Conv_PDF(ByVal Path As String, ByVal Fn As String)
43 Dim filePath As String, objOffice As Object
44 filePath = Path & "\PDF" & Left$(Fn, InStrRev(Fn, ".")) & "pdf"
45 Path = Path & Fn
46 Select Case Get_Extension(Fn) 'ファイル名から拡張子を取得
47 Case "xls", "xlsx"
48 Set objOffice = Excel.Application
49 With objOffice.Workbooks.Open(Path)
50 .ExportAsFixedFormat Type:=xlTypePDF, _
51 Filename:=filePath, Openafterpublish:=False
52 .Close
53 End With
54 Case "doc", "docx"
55 Set objOffice = CreateObject("Word.Application")
56 With objOffice.Documents.Open(Path)
57 .ExportAsFixedFormat OutputFileName:=filePath, _
58 ExportFormat:=17
59 .Close
60 End With
61 objOffice.Quit
62 Case "ppt", "pptx"
63 Set objOffice = CreateObject("Powerpoint.Application")
64 With objOffice.Presentations.Open(Path)
65 .SaveAs Filename:=filePath, FileFormat:=32
66 .Close
67 End With
68 objOffice.Quit
69 End Select
70End Sub
71
PDF化コードの使い方
ここでは、上記のコードの簡単な使い方を解説します。下記の要領で、特定のフォルダ内のすべてのファイルをPDF化します。
新規フォルダを作成し、PDF化したいファイルだけをすべて格納してください。
任意の方法でマクロを起動してください。
マクロを起動するとファイルダイアログが開くので、STEP1で生成したフォルダを選択してください。
選択したフォルダの下層に「PDF」フォルダが生成され、PDF化したファイルが格納されています。
PDF化コードの解説
さて、使い方も分かったところで、上記のコードの詳細について、各パーツごとに解説します。
可能であれば、コード全体と見比べながら読み進めてください。
パーツ①メインのSubプロシージャ
PDF化コードは、Subプロシージャである「Convert_to_PDF()」をメインに、他のプロシージャを呼び出す構造となっています。
1Sub Convert_to_PDF()
2 Dim strDirPath As String
3 strDirPath = Search_Directory() 'フォルダの選択
4 If Len(strDirPath) = 0 Then Exit Sub
5 Call Make_Dir(strDirPath, "\PDF") 'フォルダ作成
6 Call Search_Files(strDirPath)
7 MsgBox ("pdfファイル作成が完了しました")
8End Sub
大まかな流れとしては、下記のとおりです。
「Search_Directory()」でstrDirPathに、PDF化対象のフォルダパスを格納
「Make_Dir()」で、PDF化したファイルを保存(出力)するためのフォルダを生成
「Search_Files()」で、指定したフォルダ内にあるファイルをPDF化します
パーツ②フォルダ選択用のプロシージャ「Search_Directory()」
この部分では、PDF化したいファイルが格納されているフォルダを指定し、フォルダパスを返します。
ここで取得したフォルダパスを、「Convert_to_PDF()」のstrDirに格納します。
1Private Function Search_Directory() As String 'フォルダの選択
2 With Application.FileDialog(msoFileDialogFolderPicker)
3 If .Show = True Then Search_Directory = .SelectedItems(1)
4 End With
5End Function
パーツ③PDF化したファイル保存用のフォルダを生成「Make_Dir()」
この部分では、「Search_Directory()」で指定したフォルダの下層に、PDF化した後のファイルを保存するためのフォルダを生成します。
1Private Sub Make_Dir(ByVal Path As String, ByVal Folder_name As String)
2 If Dir(Path & Folder_name, vbDirectory) = "" Then 'フォルダの存在確認
3 MkDir Path & Folder_name 'フォルダ作成
4 End If
5End Sub
パーツ④ファイルを検索してヒットすればPDF化「Search_Files()」
この部分では、下記の手順で指定したフォルダ内のファイル全て検索し、PDF化します。
指定したフォルダ内に、自ファイル(Excel)以外のファイルがあるか確認
ファイルを発見したときには、「Conv_PDF()」でファイルをPDF化
PDF化が終わったら、指定したフォルダ内に他のファイルがないか確認。
STEP3で他のファイルが見つからなくなるまで繰り返し
1Private Sub Search_Files(ByVal Path As String)
2 Dim strFile As String, strET As String
3 strFile = Dir(Path & "\" & "*.*") 'ファイルの存在確認
4 Application.ScreenUpdating = False
5 Do Until strFile = ""
6 If ThisWorkbook.FullName <> Path & "\" & strFile Then
7 Call Conv_PDF(Path, "\" & strFile)
8 End If
9 strFile = Dir() '次のファイル確認
10 Loop
11 Application.ScreenUpdating = True
12End Sub
パーツ⑤ファイルを1つ1つPDF化「Conv_PDF」
この部分では、ファイルの拡張子によって条件分岐し、それぞれPDF化を行います。
1Private Sub Conv_PDF(ByVal Path As String, ByVal Fn As String)
2 Dim filePath As String, objOffice As Object
3 filePath = Path & "\PDF" & Left$(Fn, InStrRev(Fn, ".")) & "pdf"
4 Path = Path & Fn
5 Select Case Get_Extension(Fn) 'ファイル名から拡張子を取得
6 Case "xls", "xlsx"
7 Set objOffice = Excel.Application
8 With objOffice.Workbooks.Open(Path)
9 .ExportAsFixedFormat Type:=xlTypePDF, _
10 Filename:=filePath, Openafterpublish:=False
11 .Close
12 End With
13 Case "doc", "docx"
14 Set objOffice = CreateObject("Word.Application")
15 With objOffice.Documents.Open(Path)
16 .ExportAsFixedFormat OutputFileName:=filePath, _
17 ExportFormat:=17
18 .Close
19 End With
20 objOffice.Quit
21 Case "ppt", "pptx"
22 Set objOffice = CreateObject("Powerpoint.Application")
23 With objOffice.Presentations.Open(Path)
24 .SaveAs Filename:=filePath, FileFormat:=32
25 .Close
26 End With
27 objOffice.Quit
28 End Select
29End Sub
うまく動作しないときの対処法
ここでは、上記のコードでうまく動作しなかったときの対処法を解説します。
FileDialog(msoFileDialogFolderPicker)が実行されない
エラーメッセージが「実行時エラー’91’ オブジェクト変数またはWithブロック変数が設定されていません。」と表示されたときは、このエラーの可能性があります。原因は「動作環境がExcel for Mac」であることが考えられます。
これは動作環境が問題なので、Windows環境で動作させるか、AppleScriptを実行する必要があります。
